Unpacking The Oasis Random Number Generation Implementation
RNGs are tools that play a crucial role in solving different problems with onchain randomness for Oasis and other public blockchains.
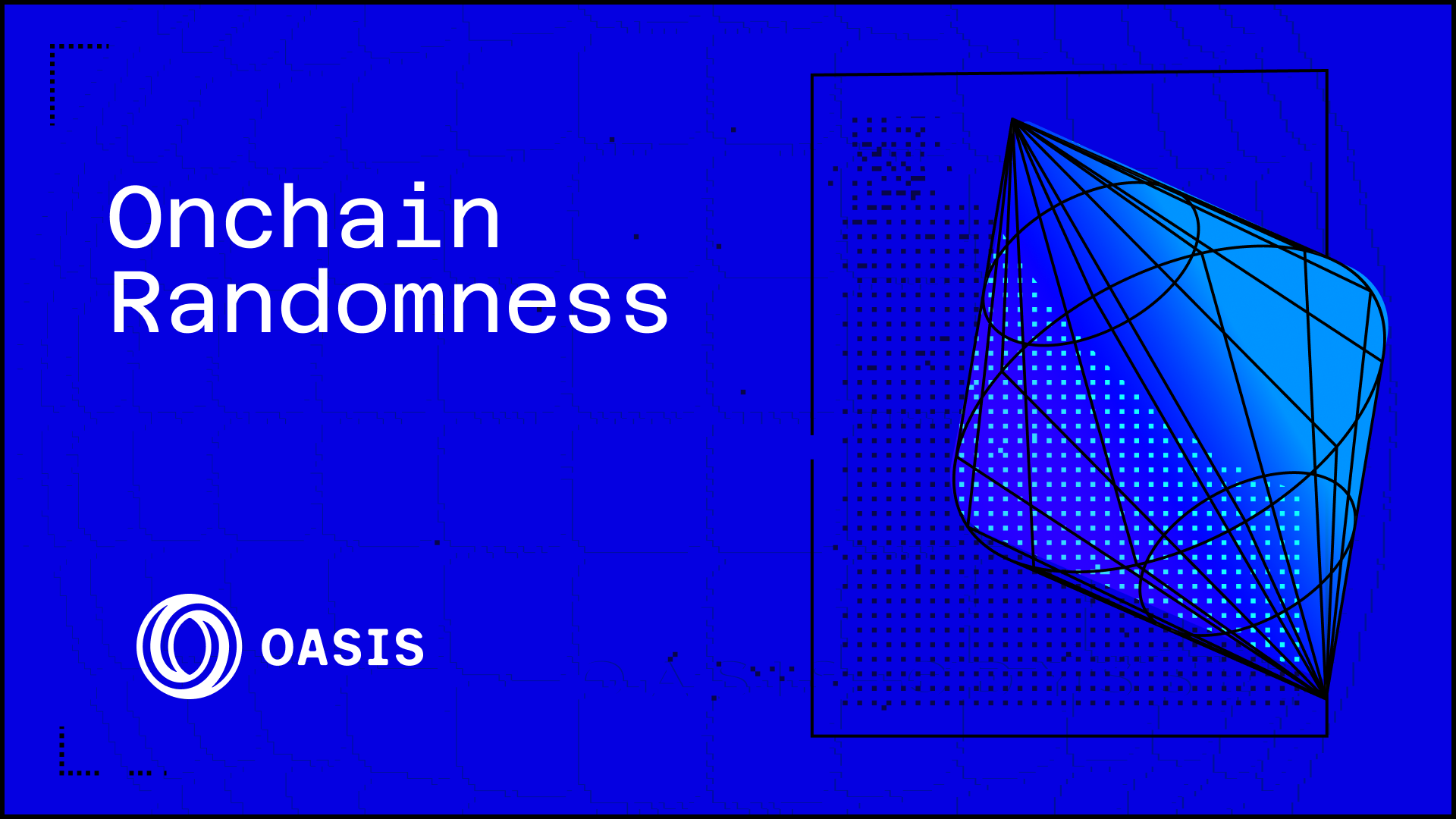
Summary:
- Random number generators (RNGs) work around the deterministic nature of computers by using external inputs or algorithms to create randomness.
- RNG is vital for dApps to ensure fairness and uniqueness, and blockchains to generate unpredictable keys, encrypt data, authenticate users, etc.
- Oasis offers developers the randomBytes precompile for easy access to secure onchain randomness generated from private ephemeral entropy by using VRFs and Merlin transcripts for domain separation
.
What is a Random Number Generator?
Programming a computer to produce random numbers is hard because computers are deterministic machines designed to follow predictable instructions, not generate randomness. To solve this, random number generators (RNGs) use external input or complex algorithms to deliver truly (or almost) random numbers.
RNGs come in two primary flavors:
- Deterministic RNG: Also known as pseudo-random number generators, this type generally uses algorithms to transform a starting "seed" value into sequences that mimic randomness, though the output is predictable if the seed is known.
- Non-deterministic RNG: This type generates completely random numbers by tapping into erratic physical phenomena, like dice rolls or photon scattering, bypassing the computer's reliance on predictable patterns.
Why Does a Blockchain Need Random Numbers?
In general, randomness is a useful tool to solve different kinds of algorithmic problems. In Web3, secure RNG is essential for dApps because its unpredictable inputs are needed for things like provable fairness in gaming, truly unique NFTs, or unbiased/tamperproof outcomes for lotteries or other selection processes.
RNG also plays a crucial role in cryptographic operations within a blockchain. It produces the unique keys or values needed for securing transactions, encrypting data, or authenticating users, ensuring that outputs cannot be guessed or replicated. If the RNG is weak or flawed, it's possible to reverse-engineer keys, manipulate outcomes, and otherwise exploit the system.
In practice, onchain randomness is tricky because blockchains use deterministic rules, and all nodes must reach a consensus. Most also do not have parameters in their trust boundary that can provide enough entropy to simulate unpredictable physical events. But the good news is that multiple workable versions of deterministic RNGs exist, e.g., verifiable random functions, commit-reveal schemes, or the Oasis method, which uses VRFs and some other cryptographic primitives.
How Does Oasis Approach RNG?
Oasis Network was built for privacy and scalability. This setup includes Sapphire, the ecosystem's confidential EVM network. Sapphire streamlines RNG through its randomBytes precompile, which provides secure randomness to developers via a simple Solidity function.
This abstraction empowers developers to integrate randomness into their applications without dealing with the complexities of blockchain RNG. This accelerates development timelines, reduces the likelihood of implementation mistakes, and contributes to innovation by making RNG more approachable.
How Does Oasis Random Number Generation Actually Work?
Generating the Per-Block Root RNG
- Confidential runtimes like Sapphire communicate with a key manager runtime to generate secret keys. The key manager supports multiple kinds of keys, some of which are long-term and some of which are ephemeral.
- The ephemeral keys are used for things like encrypting transactions and also the RNG. To improve security, these keys are never persisted anywhere and are rotated each epoch. This means fresh keys are generated, and after a while, old keys get securely erased and cannot be recovered even if any component is later compromised.
- The RNG exposed to Sapphire contracts is initialized on every block. Each block uses private ephemeral entropy obtained from the key manager runtime. Only remotely attested Sapphire instances can obtain this private entropy inside the TEE, which ensures that no external observers can learn anything about the RNG state.
- Next, this entropy is processed and used as a root Verifiable Random Function (VRF) key. To turn this entropy into a usable key, Sapphire employs SHA-3-derived algorithms like TupleHash, KMAC256, and cSHAKE. These functions process the epoch-specific entropy from the key manager runtime, producing a unique per-block root RNG that anchors all subsequent randomness in the block with consistency and security.
Domain Separation for Per-Transaction RNGs
- The per-block root RNG alone isn’t enough. Each transaction needs its own private RNG, which is where domain separation comes in. Sapphire builds on the key manager’s output by using Merlin transcripts to initialize per-transaction RNGs from the root RNG, customizing randomness for individual interactions using transaction-specific data.
- This customization is implemented in the Rust Runtime SDK used to build Sapphire, which handles the VRF and domain separation schemes. Together with the key manager’s private entropy, it ensures private, unbiased, and unpredictable outputs are exposed to developers via the randomBytes precompile. More here.
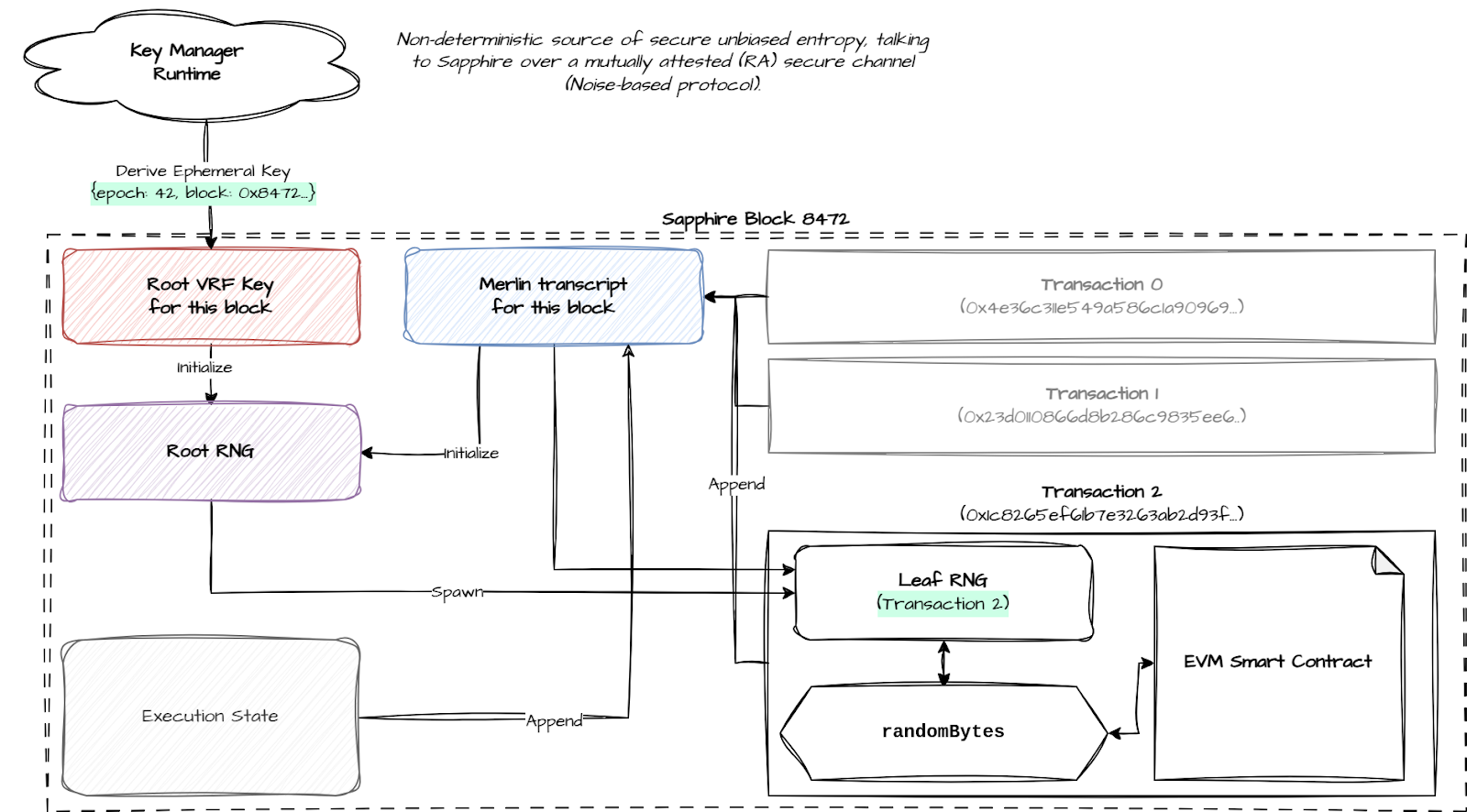
Code Examples
Basic Random Number Generation
This snippet shows how to generate a 32-byte random value, ideal for straightforward RNG needs in a dApp like a poker game.
Random Number for Signing Key Pair
This snippet uses randomBytes to seed a signing key pair generation, which could be part of a more complex RNG-driven mechanic while ensuring cryptographic security.
If you're building an application that requires secure random number generation, click here and reach out to Oasis contributors via Discord, Telegram, or other social channels if you have questions about leveraging this innovative solution.